Substring function in C# helps you to retrieve a part of the string. It means you can extract a specific portion of a given string like retrieving first n characters, last n characters or characters in the middle of string specified as position.
The method can be used in the below given overloaded forms.
1: String.Substring(Int32) Method
2: String.Substring(Int32, Int32) Method
1: String.Substring(Int32) Method: We use String.Substring(startIndex) method to retrieve a substring from the specified position as “startIndex” parameter to the end of the string. The passed parameter “startIndex” specifies the start position of the substring and then continues to the end of the string. We can understand it in a better way by the following example.
string sampleStr = "CodeForDevs";
// retrieves the substring from index 4
Console.WriteLine(sampleStr.Substring(4));
// retrieves the substring from index 7
Console.WriteLine(sampleStr.Substring(7));
Output:
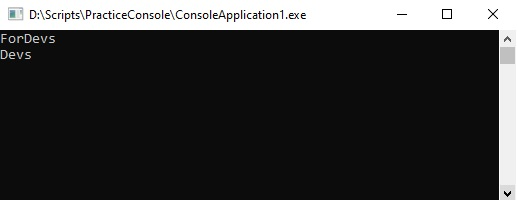
First character in a string starts at 0th position. Substring starts characters index counting index from 0 and returns “ForDevs” from index 4 and “Devs” from index 7.
C | o | d | e | F | o | r | D | e | v | s |
0 | 1 | 2 | 3 | 4 | 5 | 6 | 7 | 8 | 9 | 10 |
2: String.Substring(Int32, Int32) Method: We use String.Substring(int startIndex, int length) method to extract a substring of specified length, described by parameter length, and starts from the specified position described by parameter startIndex.
string sampleStr = "CodeForDevs";
// retrieves the substring from index 0 to length 7
Console.WriteLine(sampleStr.Substring(0, 7));
// retrieves the substring from index 4 to length 6
Console.WriteLine(sampleStr.Substring(4, 6));
Output:
CodeFor
ForDev
C# Substring function Examples
Retrieving first n characters from a given string:
The below-given code snippet retrieves first n characters from the given string. You need to replace n with the number of characters you want to get.
For example, if you need to extract first 11 characters from the given string.
string GivenStr = "CodeForDevs.com";
// retrieves the first 11 characters
Console.WriteLine(GivenStr.Substring(0, 11));
Output:
CodeForDevs
Retrieving last n characters from a given string:
The below-given code snippet retrieves the last n characters from the given string. You need to replace n with the number of characters you want to get.
For example, if you need to extract the last 4 characters from the given string.
string GivenStr = "CodeForDevs.com";
int startIndex = GivenStr.Length - 4;
// retrieves the last 4 characters
Console.WriteLine(GivenStr.Substring(startIndex, 4));
Output:
.com