This article will help you to know how to export C# data table to HTML. Suppose below given data is in CSV format and you need to generate the code HTML code for that data.
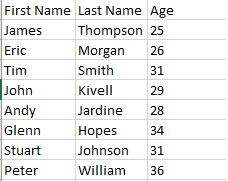
Step1: Define the function to store that csv data to C# data table object dt. You can refer to my previous post Convert CSV file to C# Data Table for better explanation of CSVtoDataTable function.
DataTable dt = CSVtoDataTable(CsvFilePath, csvDelimiter);
Step2: Define the function to convert Data table to HTML. Use the below given code snippet directly in your program to export ExportDatatableToHtml. This code can be used to every data table to HTML. You can customize the format HTML table style as per your requirement.
public string ExportDatatableToHtml(DataTable dt)
{
StringBuilder strHTML = new StringBuilder();
strHTML.Append("<html >");
strHTML.Append("<head>");
strHTML.Append("<style>");
strHTML.Append("#customers { font-family: cambria; border-collapse: collapse; width: auto;}");
strHTML.Append("#customers td, #customers th { border: 1px solid #ddd; padding: 8px; }");
strHTML.Append("#customers tr:nth-child(even){background-color: #f2f2f2;}");
//strHTML.Append("#customers tr:hover {background-color: #ddd;}");
strHTML.Append("#customers th { padding-top: 12px; padding-bottom: 12px; text-align: left; background-color: #4CAF50; color: white;}");
strHTML.Append("</style>");
strHTML.Append("</head>");
strHTML.Append("<body>");
strHTML.Append("<table id= \"customers\" >");
strHTML.Append("<tr >");
foreach (DataColumn myColumn in dt.Columns)
{
strHTML.Append("<th ><b>");
strHTML.Append(myColumn.ColumnName);
strHTML.Append("</th></b>");
}
strHTML.Append("</tr>");
foreach (DataRow myRow in dt.Rows)
{
strHTML.Append("<tr >");
foreach (DataColumn myColumn in dt.Columns)
{
strHTML.Append("<td >");
strHTML.Append(myRow[myColumn.ColumnName].ToString());
strHTML.Append("</td>");
}
strHTML.Append("</tr>");
}
strHTML.Append("</table>");
strHTML.Append("</body>");
strHTML.Append("</html>");
string Htmltext = strHTML.ToString();
return Htmltext;
}
Step3: Call the function ExportDatatableToHtml: The below code snippet calls the function ExportDatatableToHtml and stores HTML code of data table in HtmlCode variable of string data type.
string HtmlCode = ExportDatatableToHtml(dt);
Step4: Export the HTML code to HTML file.
System.IO.File.WriteAllText(@"D:\TEST\Sample.HTML", HtmlCode);
At the specified path, you will get the Sample.HTML file. Double clicking that html file, you will get table as shown below.
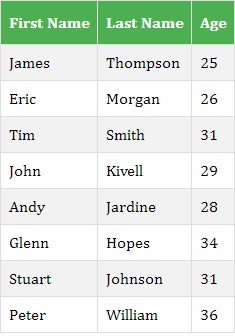
In the above code example, we stored csv data to data table then we exported the HTML code of data table. We can use the same code to convert data table to HTML if data table is populated programmatically or data exported from database table.