This article demonstrates the C# code snippet to easily convert excel files to csv files. In this code example, you can define your own desired csv delimiter (comma, semicolon, pipe etc.)
To do so, you need to use a third party .Net library Spire.xlsx that is free of cost library for .XLSX file conversion to .CSV.
You can download this free library from this link.
You can add this library directly into your C# code program using Manage Nuget Package Manager in Visual Studio.
Once you have added this library to your project, it will show in references group as Spire.XlS.
Below given step by step code snippet will guide you to convert excel file to csv.
Step 1: You need to add reference of this library by below code.
using Spire.Xls;
using System.Text;
Step 2: Declare the variable to hold the path of CSV file on which CSV file will be exported and delimiter of CSV file.
string FilePath = @"D:\TEST\Sample_Data.xlsx";
char csvDelimiter = ';';
Step 3: Define the function as below.
public void SpireXlsxToCSV(string fpath, ,char csvDelimiter)
{
//Create a Workbook object
Workbook wb = new Workbook();
//Load a xls or xlsx Excel file
wb.LoadFromFile(fpath);
//Get a specified worksheet
Worksheet sht = wb.Worksheets[0];
//Save the worksheet as CSV
sht.SaveToFile(fpath.Replace(".xlsx",".csv"), csvDelimiter, Encoding.UTF8);
}
Output:
When you run the code, you will see that a csv file is generated on the same location with the name Sample_Data.csv
XLSX Data
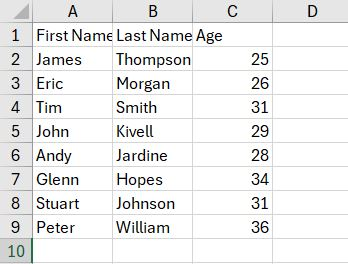
CSV Data after conversion
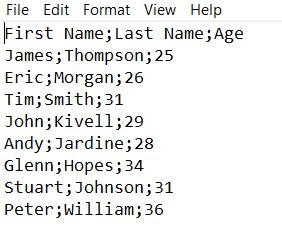