This post shows an example of listing name of all files with extension, available in a folder. We need to import System.IO namespace in our program and Directory.GetFiles() method helps you to get the list of all files available in the specified folder.
For example, we take a folder “Sample Data” in D drive which contains csv files as shown in below snap.
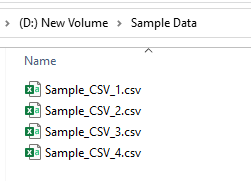
In order to list all the files available in this folder we write the below given C# program.
using System;
using System.IO;
namespace DirectoryExample
{
class Program
{
static void Main(string[] args)
{
string path = @"D:\Sample Data\";
string[] fileList = Directory.GetFiles(path);
foreach (string fl in fileList)
{
FileInfo fi = new FileInfo(fl);
Console.WriteLine(fi.Name);
}
Console.Read();
}
}
}
Output:
Sample_CSV_1.csv
Sample_CSV_2.csv
Sample_CSV_3.csv
Sample_CSV_4.csv
In the above C# code, Directory.GetFiles() method returns the list of files on path “D:\Sample Data\” and stores in fileList array and then by the help of Name property of object of FileInfo, we iterate to all file names printing by foreach loop.