In this post, we will demo an example of adding a CheckBox Column in DataGridView control in Windows Form application using C#. In this example, you will get to know how to read selected rows of DataGridView. Suppose that we have below sample data in a datatable dT.
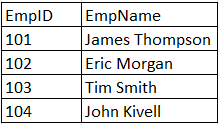
First we will bind this datatable data to DataGridView using below code.
dataGridView1.DataSource = dT;
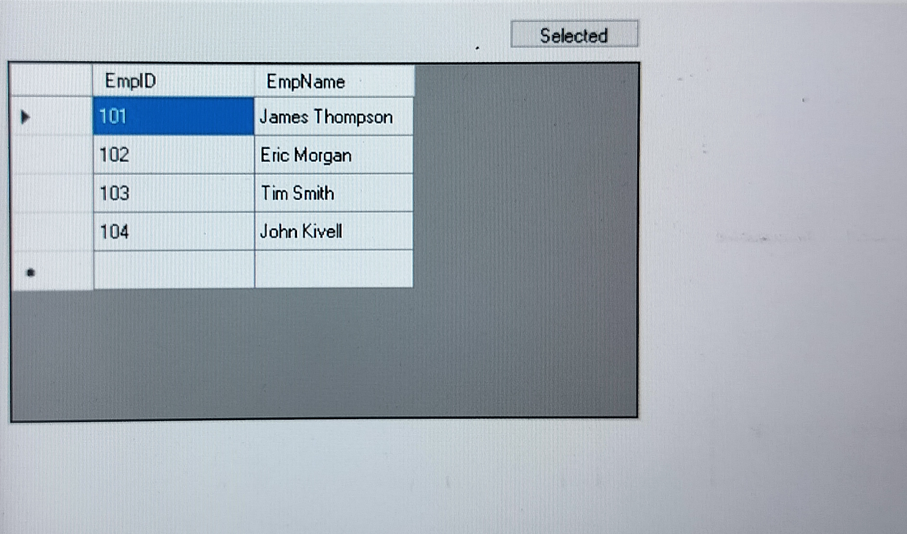
Now we will add a checkbox column in DGV using below C# code snippet.
DataGridViewCheckBoxColumn checkBoxColumn = new DataGridViewCheckBoxColumn();
checkBoxColumn.HeaderText = ""
checkBoxColumn Width = 50;
checkBoxColumn.Name = "Select";
dataGridView1.Columns.Insert(0, checkBoxColumn);
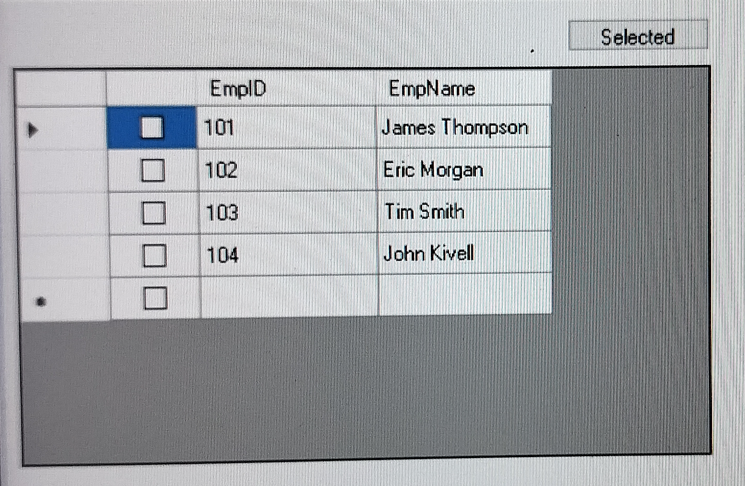
Now we have added a check box in DataGridView of a Win Form application developed in C#. User can check or uncheck required checkbox as per his requirement.
Get the Selected Rows in DataGridView:
Below given C# code snippet helps you get the rows selected in DataGridView. You need to write this code snippet in click event of Selected button.
private void btnSelested_Click(object sender, EventArgs e)
{
foreach (DataGridViewRow row in dataGridView1.Rows)
{
bool isSelected = Convert.ToBoolean(row.Cells["Select"].Value);
if (isSelected)
{
string selection = Convert.ToString(row.Cells("EmpName"].Value);
MessageBox.Show("Selected Rows:" selection);
}
}
}
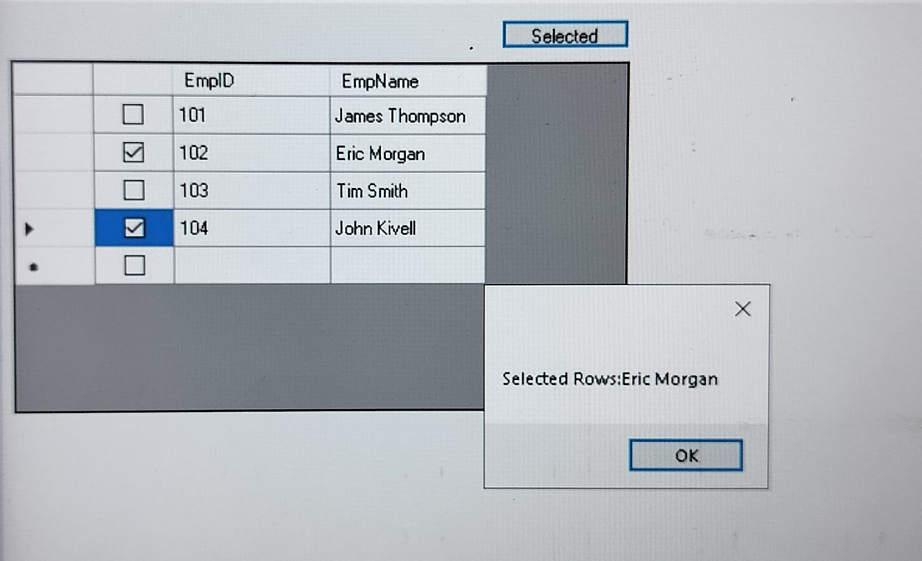
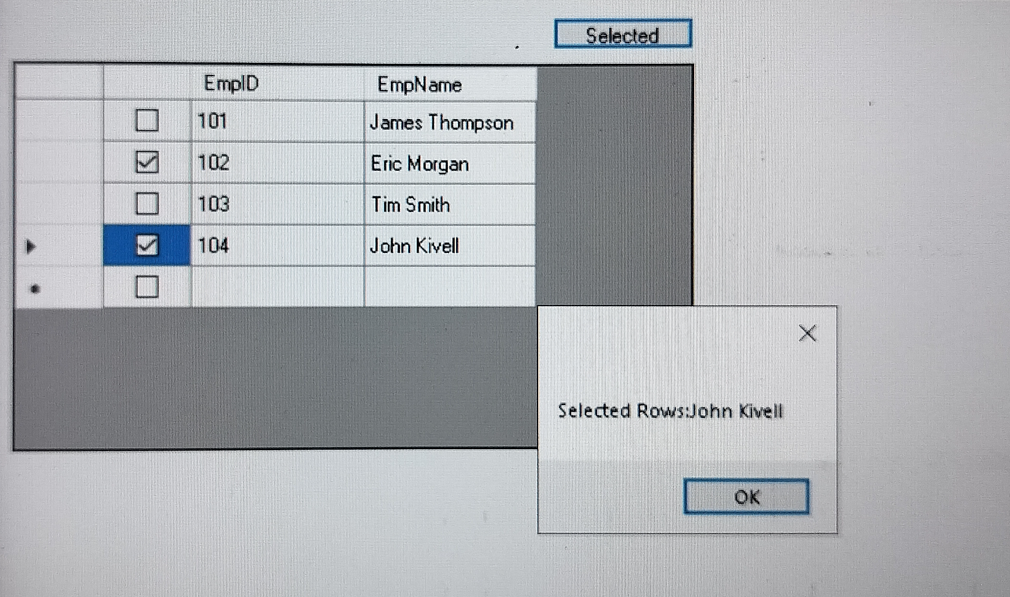
Check/Uncheck or select all checkbox in DataGridView in C# Windows Application:
You may also need to check or check all the checkboxes of DataGridView. To do this, you need to add a checkbox control in your application and write below given code in CheckedChange event handler of check box. Now when Select All check box is checked, it will check all the checkboxes and unchecking Select All checkbox, you can uncheck all the checkboxes.
private void checkBox1_CheckedChanged(object sender, EventArgs e)
{
if(checkBox1.Checked)
{
foreach (DataGridViewRow row in this dataGridView1.Rows)
{
row.Cells["Select"].Value = true;
}
}
else
{
foreach (DataGridViewRow row in this dataGridView1.Rows)
{
row.Cells["Select"].Value = false;
}
}
}
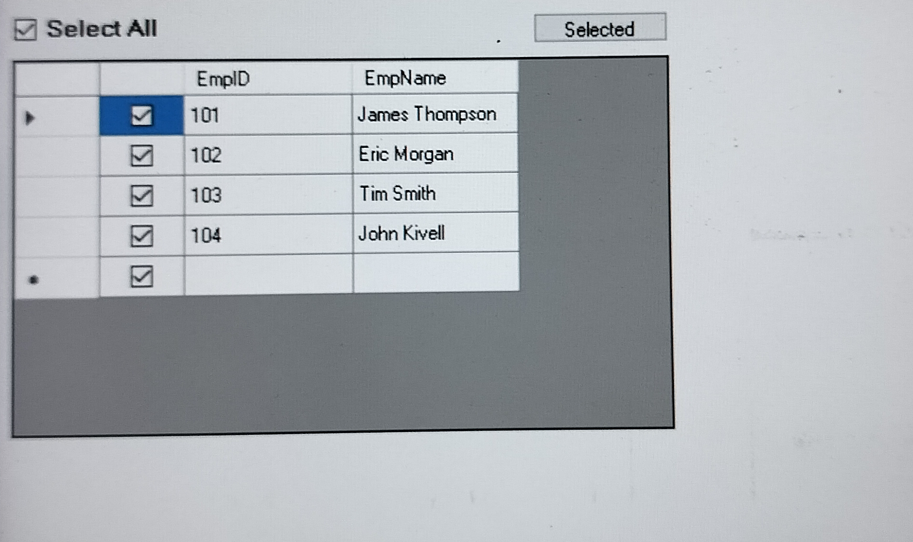